HOW TO HANDLE LOCALIZATION FROM A CLIENT APPLICATION IN DOTNET CORE WEB API
29.04.2021How to use localization in dotnet core?
var supportedCultures = new[] { new CultureInfo("de-CH"), new CultureInfo("en-UK") }; app.UseRequestLocalization(new RequestLocalizationOptions { DefaultRequestCulture = new RequestCulture(new CultureInfo("de-CH")), SupportedCultures = supportedCultures, SupportedUICultures = supportedCultures, });
The dotnet filter pipeline
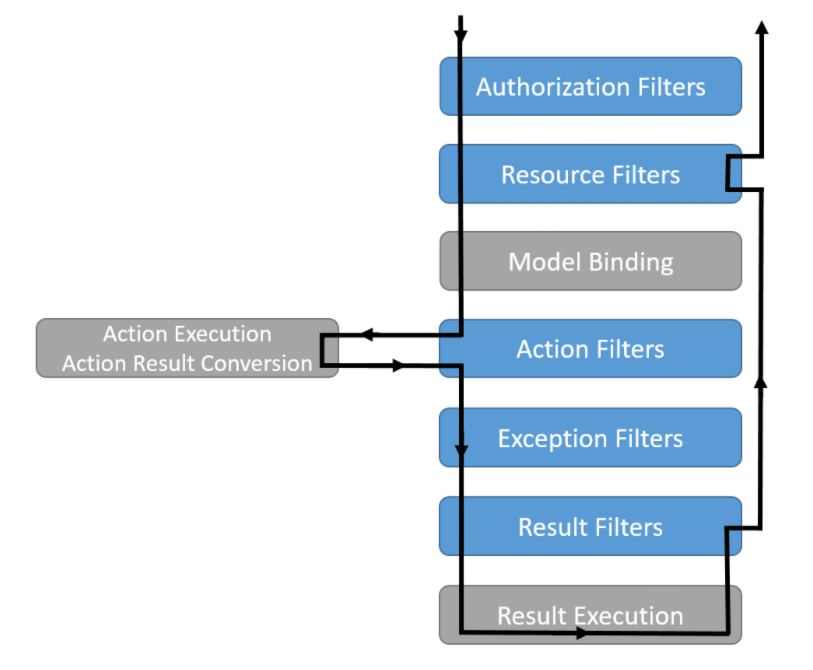
Backend implementation
public class LanguageFilter : IResourceFilter { public void OnResourceExecuting(ResourceExecutingContext) { if (context.HttpContext.Request.Headers.ContainsKey("AcceptLanguage") && context.HttpContext.Request.Headers["AcceptLanguage"].Count > 0) { LanguageHelper.SetLanguage(context.HttpContext.Request.Headers["AcceptLanguage"].First()); } } public void OnResourceExecuted(ResourceExecutedContext) { } }
public static class LanguageHelper { public static void SetLanguage(string language) { var culture = new CultureInfo("de-CH"); if (language == "en-UK" || language.Contains("en")) { culture = new CultureInfo("en-UK"); } Thread.CurrentThread.CurrentCulture = culture; Thread.CurrentThread.CurrentUICulture = culture; } }
Frontend implementation
@Injectable() export class HeaderInterceptor implements HttpInterceptor { constructor(private translationService: TranslationService) {} intercept(request: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> { return this.authService.getTokenSilently$().pipe( mergeMap(token => { request = request.clone({ setHeaders: { 'Access-Control-Allow-Origin': '*', 'Access-Control-Allow-Method': 'GET,POST,OPTIONS,DELETE,PUT,PATCH', 'Content-Type': 'application/json; charset=utf-8', 'Cache-Control': 'no-cache', Accept: 'application/json', AcceptLanguage: this.translationService.getLanguageString(), Pragma: 'no-cache' } }); return next.handle(request); }), catchError(err => throwError(err)) ); } }
var subject = AppStrings.GuestSubscription;