Setup sentry application monitoring in an angular universal project
01.12.2021Getting started
npm install --save @sentry/angular @sentry/tracing
Configuration
import { enableProdMode } from "@angular/core"; import { platformBrowserDynamic } from "@angular/platform-browser-dynamic"; import * as Sentry from "@sentry/angular"; import { Integrations } from "@sentry/tracing"; import { AppModule } from "./app/app.module"; Sentry.init({ dsn: "https://...." , integrations: [ // Registers and configures the Tracing integration, // which automatically instruments your application to monitor its // performance, including custom Angular routing instrumentation new Integrations.BrowserTracing({ tracingOrigins: ["localhost", "https://yourserver.io/api"], routingInstrumentation: Sentry.routingInstrumentation, }), ], // Set tracesSampleRate to 1.0 to capture 100% // of transactions for performance monitoring. // We recommend adjusting this value in production tracesSampleRate: 1.0, }); platformBrowserDynamic() .bootstrapModule(AppModule) .then(success => console.log('Bootstrap success')) .catch(err => console.error(err));
providers: [ { provide: Sentry.TraceService, deps: [Router], }, { provide: APP_INITIALIZER, useFactory: () => () => {}, deps: [Sentry.TraceService], multi: true, }, ],
Changes in angular universal
import { isPlatformBrowser } from '@angular/common'; import { ErrorHandler, Inject, Injectable, PLATFORM_ID } from '@angular/core'; import { Integrations } from '@sentry/tracing'; import * as Sentry from '@sentry/angular'; import { environment } from '../environments/environment'; @Injectable() export class ErrorLogger implements ErrorHandler { constructor(@Inject(PLATFORM_ID) private readonly platformId: any) { if(isPlatformBrowser(this.platformId)) { Sentry.init({ dsn: '...', release: environment.sentry.environment, integrations: [ new Integrations.BrowserTracing({ tracingOrigins: ['...', '...'], routingInstrumentation: Sentry.routingInstrumentation, }), ], tracesSampleRate: 1.0, }); } } handleError(error: any): void { if (isPlatformBrowser(this.platformId)) { const eventId = Sentry.captureException(error.originalError || error); Sentry.showReportDialog({ eventId }); } } }
providers: [{ provide: ErrorHandler, useClass: ErrorLogger, deps: [] }, { provide: Sentry.TraceService, deps: [Router], }, { provide: APP_INITIALIZER, useFactory: () => (): any => { }, deps: [Sentry.TraceService], multi: true, } ],
Publish sentry release using github actions
steps: - uses: actions/checkout@v2 - name: Use Node.js ##matrix.node-version uses: actions/setup-node@v1 with: node-version: ##matrix.node-version - name: npm install, build run: | npm install npm run build:ssr - uses: azure/docker-login@v1 with: login-server: ##env.AZURE_CONTAINER_URL username: ##secrets.AZURE_CONTAINER_USERNAME password: ##secrets.AZURE_CONTAINER_PASSWORD - name: Build the Docker image working-directory: ./ run: | docker build . --file Dockerfile --tag ##env.AZURE_CONTAINER_URL/##env.BM_TAG docker push ##env.AZURE_CONTAINER_URL/##env.BM_TAG - name: Login via Az module uses: azure/login@v1.1 with: creds: ##secrets.AZURE_BM_CREDENTIALS enable-AzPSSession: false - name: Deploy Azure WebApp uses: Azure/webapps-deploy@v2 with: app-name: ##env.AZURE_BM_WEBAPP_NAME images: ##env.AZURE_CONTAINER_URL/##env.BM_TAG - name: Create Sentry release uses: getsentry/action-release@v1 env: SENTRY_AUTH_TOKEN: ## secrets.SENTRY_AUTH_TOKEN SENTRY_ORG: ##secrets.SENTRY_ORG SENTRY_PROJECT: ##secrets.SENTRY_PROJECT with: environment: prod, set_commits: auto sourcemaps: dist/
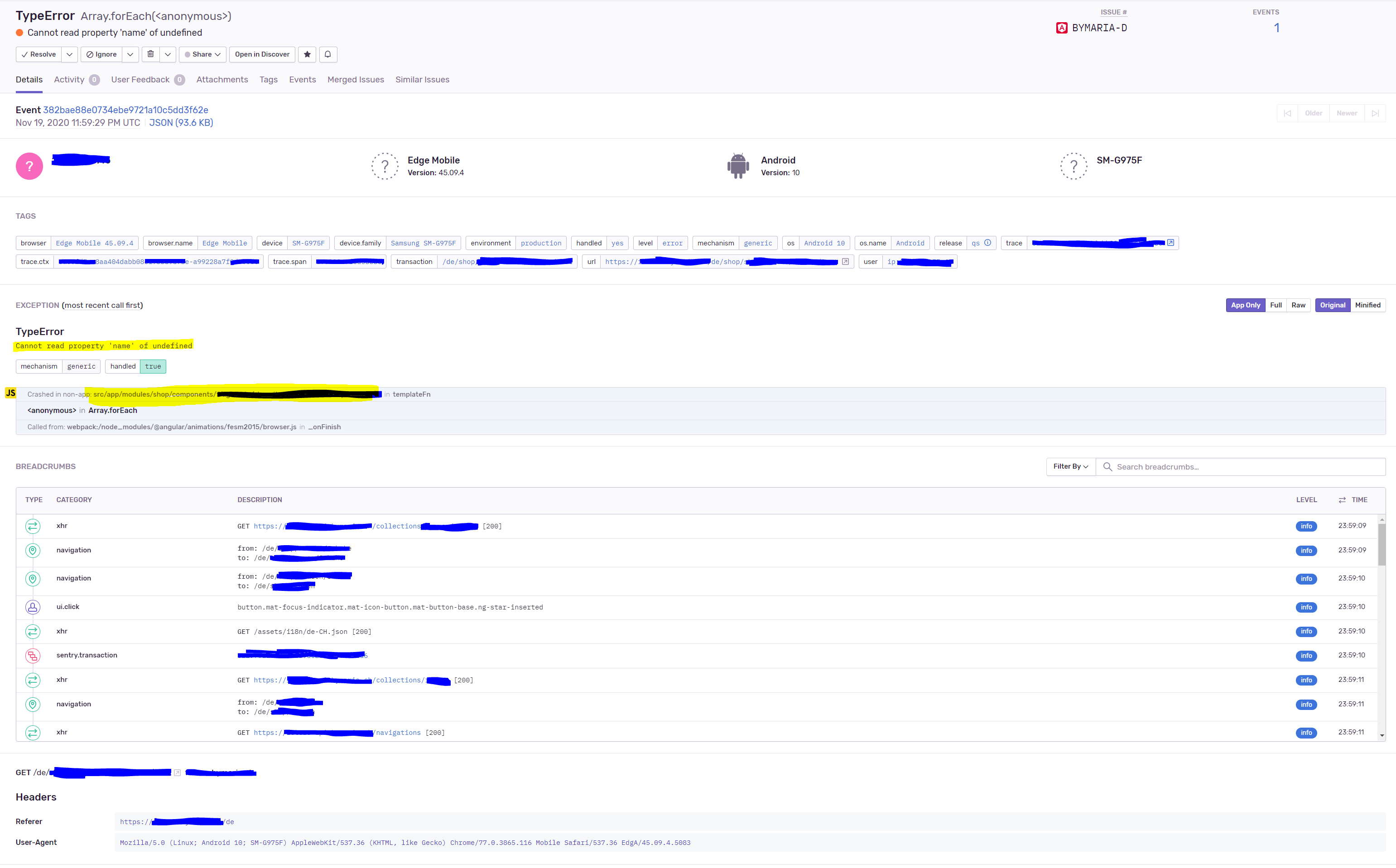